react-router-dom v4版路由及传参
日期:2018-09-14
来源:程序思维浏览:3335次
现在最新版本的react路由是react-router-dom,那么他如何使用呢?下面由我来给大家分享一下:
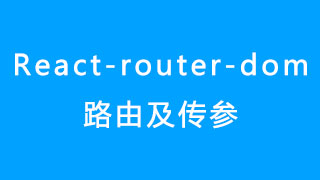
1、安装依赖 不需要指定版本,默认最新
npm i react-router react-router-dom --save-dev
2、引入主要组件对象
import {BrowserRouter as Router,Route,Link,Redirect,Switch} from 'react-router-dom'
3、书写结构在组件内部,不需要写在render中,Router组件有且只有一个根节点,除了路由组件,可以写入其他标签
默认 Route所在的位置为路由组件显示的容器(tips:Link写在Router内部形成路由结构)
<Router>
<div>
<Link to="/home">首页</Link>
<Link to="/about">关于</Link>
<Route path="/home" component={Home}></Route>
<Route path="/about" component={About}></Route>
</div>
</Router>
4、路由重定向 通过 Redirect组件对象,设置to属性
<Redirect to="/about"/>
5、路由参数传递
/a/1 ---this.props.match.params.id
/a?id=1---this.props.location.query.id
6、事件中进行路由切换跳转
this.props.history.push('/home')
因为BrowserRouter 相当于 <Router history={history}>故可直接通过history进行push跳转
高版嵌套路由写法:
<Router>
<div>
{
this.state.arr.map(function(item,i){
return(
<div key={i}>
<Link to={{pathname:'/detail',query:{id:item.pid}}}>{item.pname}</Link>
</div>
)
})
}
<hr/>
<Route path="/detail" component={Detail}></Route>
</div>
</Router>
然后在所导入的组件引入import Detail from "./detail"
7、钩子函数:
componentWillReceiveProps(a)可时时更新传入的新数据,相当于vue的watch( )监听
componentDidMount( ) 接收id并请求数据,但页面数据只能刷新一次
import React from 'react';
import $ from 'jquery'
class Detail extends React.Component{
constructor(props){
super(props)
this.state={
detail:''
}
}
render(){
return(
<div>
<h2>详情</h2>
<p>{this.state.detail}</p>
</div>
)
}
//可时时更新传入的新数据,相当于vue的watch()监听
componentWillReceiveProps(a){
var _this=this;
$.ajax({
type:"get",
url:"http://jx.xuzhixiang.top/ap/api/detail.php",
data:{id:a.location.query.id},
dataType:'json',
async:true,
success:function(data){
_this.setState({detail:data.data.pdesc})
}
});
}
//接收id并请求数据,但页面数据只能刷新一次
componentDidMount(){
var _this=this;
$.ajax({
type:"get",
url:"http://jx.xuzhixiang.top/ap/api/detail.php",
data:{id:_this.props.location.query.id},
dataType:'json',
async:true,
success:function(data){
_this.setState({detail:data.data.pdesc})
}
});
}
}
export default Detail;
完整代码
app.js 高版本路由配置
import React, { Component } from 'react';
import {BrowserRouter as Router,Route,Link,Redirect,Switch} from 'react-router-dom'
import Home from './components/home'
import About from './components/about'
import Other from './components/other'
class App extends Component {
render() {
return (
<div className="App">
<h1>高版路由</h1>
<Router>
<div> {/* 有一个根节点 */}
<Link to="/home">首页</Link>
<Link to="/about">关于</Link>
<Link to="/other">其他</Link>
<Switch> {/* 解决控制台一片红色问题 */}
<Route path="/home" component={Home}></Route>
<Route path="/about" component={About}></Route>
<Route path="/other" component={Other}></Route>
<Redirect to="/home"/> {/* 路由重定向 */}
</Switch>
</div>
</Router>
</div>
);
}
}
export default App;
home.js
import React from 'react';
import {BrowserRouter as Router,Route,Link,Redirect,Switch} from 'react-router-dom'
import $ from 'jquery'
import Detail from './detail'
class Home extends React.Component{
constructor(props){
super(props)
this.state={
arr:[]
}
}
tap(){
this.props.history.push('/other')
}
render(){
return(
<div>
<h1> shouye </h1>
<button onClick={this.tap.bind(this)}>跳转other</button>
<Router>
<div>
{
this.state.arr.map(function(item,i){
return(
<div key={i}>
<Link to={{pathname:'/detail',query:{id:item.pid}}}>{item.pname}</Link>
</div>
)
})
}
<hr/>
<Route path="/detail" component={Detail}></Route>
</div>
</Router>
</div>
)
}
componentWillMount(){
var _this=this;
$.ajax({
type:"get",
url:"http://jx.xuzhixiang.top/ap/api/productlist.php",
dataType:'json',
async:true,
success:function(data){
_this.setState({arr:data.data})
}
});
}
}
export default Home;
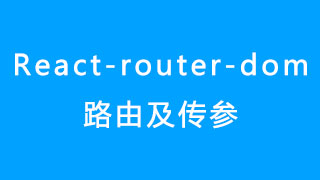
1、安装依赖 不需要指定版本,默认最新
npm i react-router react-router-dom --save-dev
2、引入主要组件对象
import {BrowserRouter as Router,Route,Link,Redirect,Switch} from 'react-router-dom'
3、书写结构在组件内部,不需要写在render中,Router组件有且只有一个根节点,除了路由组件,可以写入其他标签
默认 Route所在的位置为路由组件显示的容器(tips:Link写在Router内部形成路由结构)
<Router>
<div>
<Link to="/home">首页</Link>
<Link to="/about">关于</Link>
<Route path="/home" component={Home}></Route>
<Route path="/about" component={About}></Route>
</div>
</Router>
4、路由重定向 通过 Redirect组件对象,设置to属性
<Redirect to="/about"/>
5、路由参数传递
/a/1 ---this.props.match.params.id
/a?id=1---this.props.location.query.id
6、事件中进行路由切换跳转
this.props.history.push('/home')
因为BrowserRouter 相当于 <Router history={history}>故可直接通过history进行push跳转
高版嵌套路由写法:
<Router>
<div>
{
this.state.arr.map(function(item,i){
return(
<div key={i}>
<Link to={{pathname:'/detail',query:{id:item.pid}}}>{item.pname}</Link>
</div>
)
})
}
<hr/>
<Route path="/detail" component={Detail}></Route>
</div>
</Router>
然后在所导入的组件引入import Detail from "./detail"
7、钩子函数:
componentWillReceiveProps(a)可时时更新传入的新数据,相当于vue的watch( )监听
componentDidMount( ) 接收id并请求数据,但页面数据只能刷新一次
import React from 'react';
import $ from 'jquery'
class Detail extends React.Component{
constructor(props){
super(props)
this.state={
detail:''
}
}
render(){
return(
<div>
<h2>详情</h2>
<p>{this.state.detail}</p>
</div>
)
}
//可时时更新传入的新数据,相当于vue的watch()监听
componentWillReceiveProps(a){
var _this=this;
$.ajax({
type:"get",
url:"http://jx.xuzhixiang.top/ap/api/detail.php",
data:{id:a.location.query.id},
dataType:'json',
async:true,
success:function(data){
_this.setState({detail:data.data.pdesc})
}
});
}
//接收id并请求数据,但页面数据只能刷新一次
componentDidMount(){
var _this=this;
$.ajax({
type:"get",
url:"http://jx.xuzhixiang.top/ap/api/detail.php",
data:{id:_this.props.location.query.id},
dataType:'json',
async:true,
success:function(data){
_this.setState({detail:data.data.pdesc})
}
});
}
}
export default Detail;
完整代码
app.js 高版本路由配置
import React, { Component } from 'react';
import {BrowserRouter as Router,Route,Link,Redirect,Switch} from 'react-router-dom'
import Home from './components/home'
import About from './components/about'
import Other from './components/other'
class App extends Component {
render() {
return (
<div className="App">
<h1>高版路由</h1>
<Router>
<div> {/* 有一个根节点 */}
<Link to="/home">首页</Link>
<Link to="/about">关于</Link>
<Link to="/other">其他</Link>
<Switch> {/* 解决控制台一片红色问题 */}
<Route path="/home" component={Home}></Route>
<Route path="/about" component={About}></Route>
<Route path="/other" component={Other}></Route>
<Redirect to="/home"/> {/* 路由重定向 */}
</Switch>
</div>
</Router>
</div>
);
}
}
export default App;
home.js
import React from 'react';
import {BrowserRouter as Router,Route,Link,Redirect,Switch} from 'react-router-dom'
import $ from 'jquery'
import Detail from './detail'
class Home extends React.Component{
constructor(props){
super(props)
this.state={
arr:[]
}
}
tap(){
this.props.history.push('/other')
}
render(){
return(
<div>
<h1> shouye </h1>
<button onClick={this.tap.bind(this)}>跳转other</button>
<Router>
<div>
{
this.state.arr.map(function(item,i){
return(
<div key={i}>
<Link to={{pathname:'/detail',query:{id:item.pid}}}>{item.pname}</Link>
</div>
)
})
}
<hr/>
<Route path="/detail" component={Detail}></Route>
</div>
</Router>
</div>
)
}
componentWillMount(){
var _this=this;
$.ajax({
type:"get",
url:"http://jx.xuzhixiang.top/ap/api/productlist.php",
dataType:'json',
async:true,
success:function(data){
_this.setState({arr:data.data})
}
});
}
}
export default Home;
精品好课